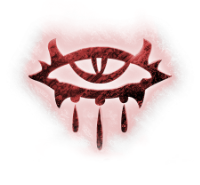 |
NWNX:EE
8193.36.12
|
|
Go to the documentation of this file.
6 const string NWNX_Core =
"NWNX_Core";
50 const string NWNX_PUSH =
"NWNXEE!ABIv2!X!Y!PUSH";
51 const string NWNX_POP =
"NWNXEE!ABIv2!X!Y!POP";
56 PlaySound(
"NWNXEE!ABIv2!" + pluginName +
"!" + functionName +
"!CALL");
61 SetLocalInt(OBJECT_INVALID, NWNX_PUSH, value);
66 SetLocalFloat(OBJECT_INVALID, NWNX_PUSH, value);
71 SetLocalObject(OBJECT_INVALID, NWNX_PUSH, value);
76 SetLocalString(OBJECT_INVALID, NWNX_PUSH, value);
81 TagEffect(value, NWNX_PUSH);
86 TagItemProperty(value, NWNX_PUSH);
91 SetLocalJson(OBJECT_INVALID, NWNX_PUSH, value);
96 return GetLocalInt(OBJECT_INVALID,
NWNX_POP);
101 return GetLocalFloat(OBJECT_INVALID,
NWNX_POP);
106 return GetLocalObject(OBJECT_INVALID,
NWNX_POP);
111 return GetLocalString(OBJECT_INVALID,
NWNX_POP);
123 return TagItemProperty(ip,
NWNX_POP);
128 return GetLocalJson(OBJECT_INVALID,
NWNX_POP);
133 string sFunc =
"PluginExists";
int NWNX_GetReturnValueInt()
Returns the specified type from the c++ side.
effect NWNX_GetReturnValueEffect()
Returns the specified type from the c++ side.
json NWNX_GetReturnValueJson()
Returns the specified type from the c++ side.
itemproperty NWNX_GetReturnValueItemProperty()
Returns the specified type from the c++ side.
float NWNX_GetReturnValueFloat()
Returns the specified type from the c++ side.
void NWNX_CallFunction(string pluginName, string functionName)
Scripting interface to NWNX.
string NWNX_GetReturnValueString()
Returns the specified type from the c++ side.
void NWNX_PushArgumentString(string value)
Pushes the specified type to the c++ side.
void NWNX_PushArgumentObject(object value)
Pushes the specified type to the c++ side.
void NWNX_PushArgumentInt(int value)
Pushes the specified type to the c++ side.
void NWNX_PushArgumentEffect(effect value)
Pushes the specified type to the c++ side.
void NWNX_PushArgumentJson(json value)
Pushes the specified type to the c++ side.
object NWNX_GetReturnValueObject()
Returns the specified type from the c++ side.
int NWNX_PluginExists(string sPlugin)
Determines if the given plugin exists and is enabled.
void NWNX_PushArgumentFloat(float value)
Pushes the specified type to the c++ side.
void NWNX_PushArgumentItemProperty(itemproperty value)
Pushes the specified type to the c++ side.